1年前には、Nexus7からbluetoothを使い、arduino経由でプラモ戦車のモーターを動かした記憶があるのだが、その時のコードを見ても全く理解できないので、すこしずつ思い出していくことに。 まともにAndroid Sudioやjavaを使ったことがない爺なので、どこまで出来ることか。
とりあえず、1年前と同じようなコードを書いてみたがBluetoothが動かない、なにか変。 結論から言うとSDKのバージョン他が違っていた。 そう言えば以前もそんな記憶が。
後は、すこしずつ継ぎ足してなんとか原型はできたが、動かしてみるとぎこちなく、とても満足できるものにはならなかった。 Sliderの使い方も分からず、やり方がまずいようだ。 これ以上は、気力も続かず一旦終了。
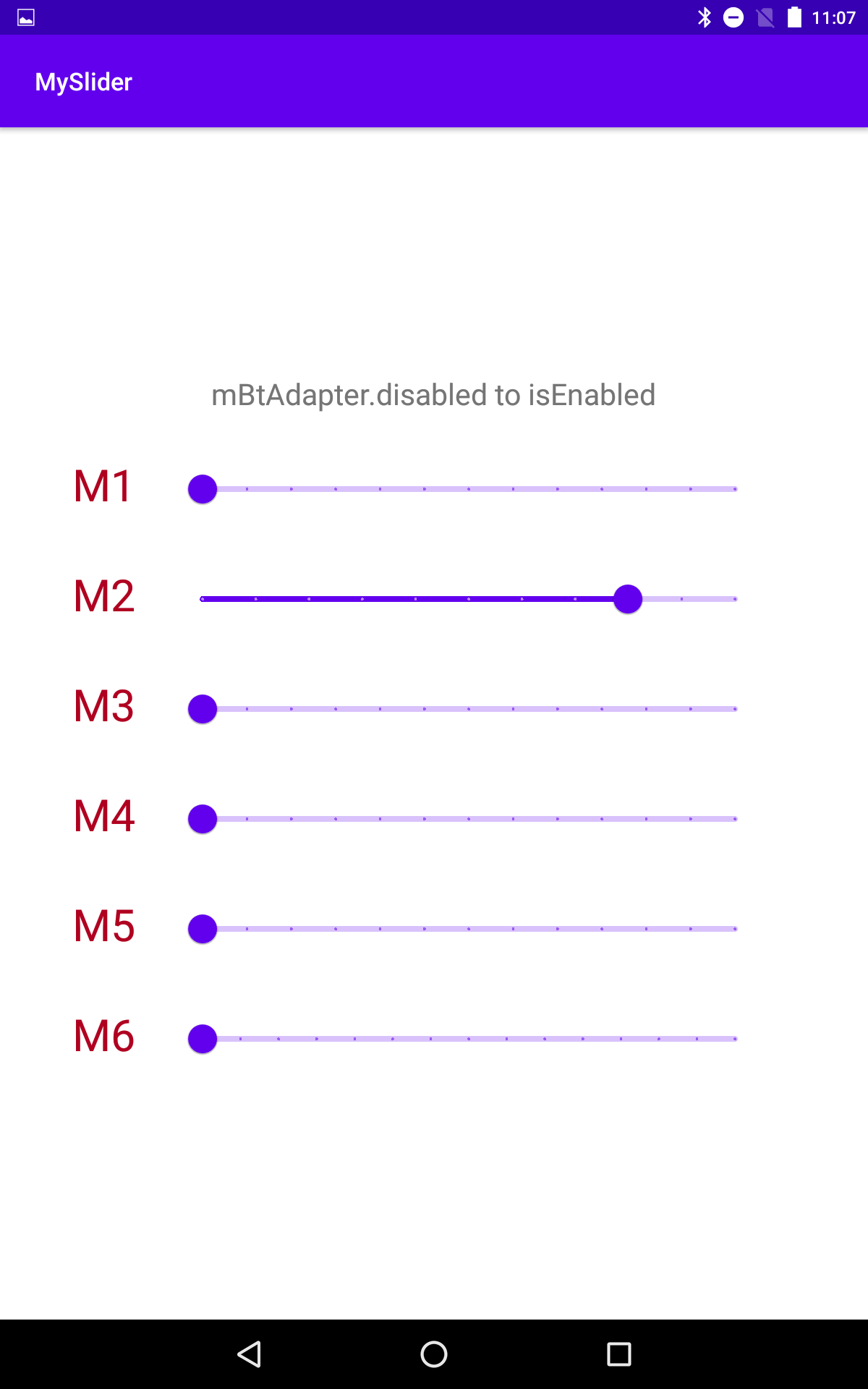
MySlider.app
build.gradle
ちなみにbuild.gradle(:app)がこちら。 targetSdkVersion を31から30に落としている。
plugins {
id 'com.android.application'
}
android {
compileSdkVersion 30
buildToolsVersion "30.0.3"
defaultConfig {
applicationId "com.example.myslider"
minSdkVersion 23
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'com.google.android.material:material:1.3.0'
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
testImplementation 'junit:junit:4.+'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
}
AndroidMainfest.xml
Android12では、さらにBluetooth権限まわりが変更となっているようだが、自分は古いままで進めることに。
なにしろNexus7はAndroid6。arduino側のドングルがClassicなので。
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myslider">
<!-- Bluetoothデバイスのスキャン機能を使用するためのパーミッションとして、
位置情報のパーミッションがAndroid 6.0から必要になった -->
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.MySlider">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
最終的に作成したMySlider.appのソースがこちら
MainActivity.java
package com.example.myslider;
import androidx.appcompat.app.AppCompatActivity;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.os.Bundle;
import java.io.IOException;
import java.io.OutputStream;
import java.util.UUID;
import android.widget.TextView;
import com.google.android.material.slider.Slider;
public class MainActivity extends AppCompatActivity {
private BluetoothDevice mBtDevice; // BTデバイス
private BluetoothSocket mBtSocket; // BTソケット
private OutputStream mOutput; // 出力ストリーム
private TextView textName;
private TextView M1textView;
private TextView M2textView;
private TextView M3textView;
private TextView M4textView;
private TextView M5textView;
private TextView M6textView;
private static final int M1=1;
private static final int M2=2;
private static final int M3=3;
private static final int M4=4;
private static final int M5=5;
private static final int M6=6;
private static String Mstrold;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Mstrold="0,135,0,0,0,0";
textName = (TextView) findViewById(R.id.displayView);
// BTの準備 --------------------------------------------------------------
// BTアダプタのインスタンスを取得
BluetoothAdapter mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
// 相手先BTデバイスのインスタンスを取得(ドングルのMacアドレスを前もって調べ入れる)
mBtDevice = mBluetoothAdapter.getRemoteDevice("0a:0a:0a:0a:0a:0a");
// BTソケットのインスタンスを取得
try {
// 接続に使用するプロファイルを指定 よく分からないがこれで繋がった
mBtSocket = mBtDevice.createInsecureRfcommSocketToServiceRecord(
UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"));
} catch (IOException e) {
e.printStackTrace();
}
// ソケットを接続する
try {
mBtSocket.connect();
mOutput = mBtSocket.getOutputStream(); // 出力ストリームオブジェクトを得る
} catch (IOException e) {
e.printStackTrace();
}
if (mBluetoothAdapter.isEnabled()) {
mBluetoothAdapter.enable();
textName.setText("mBtAdapter.disabled to isEnabled");
}
Send(M1,0); //初期状態へ
M1textView = findViewById(R.id.M1textView);
M2textView = findViewById(R.id.M2textView);
M3textView = findViewById(R.id.M3textView);
M4textView = findViewById(R.id.M4textView);
M5textView = findViewById(R.id.M5textView);
M6textView = findViewById(R.id.M6textView);
Slider sliderM1 = findViewById(R.id.M1slider);
Slider sliderM2 = findViewById(R.id.M2slider);
Slider sliderM3 = findViewById(R.id.M3slider);
Slider sliderM4 = findViewById(R.id.M4slider);
Slider sliderM5 = findViewById(R.id.M5slider);
Slider sliderM6 = findViewById(R.id.M6slider);
sliderM1.addOnChangeListener((slider1, value, fromUser) -> {
M1textView.setText(String.valueOf(value));
Send(M1,(long) value);
});
sliderM2.addOnChangeListener((slider1, value, fromUser) -> {
M2textView.setText(String.valueOf(value));
Send(M2,(long) value);
});
sliderM3.addOnChangeListener((slider1, value, fromUser) -> {
M3textView.setText(String.valueOf(value));
Send(M3,(long) value);
});
sliderM4.addOnChangeListener((slider1, value, fromUser) -> {
M4textView.setText(String.valueOf(value));
Send(M4,(long) value);
});
sliderM5.addOnChangeListener((slider1, value, fromUser) -> {
M5textView.setText(String.valueOf(value));
Send(M5,(long) value);
});
sliderM6.addOnChangeListener((slider1, value, fromUser) -> {
M6textView.setText(String.valueOf(value));
Send(M6,(long) value);
});
}
private void Send(int mno,long Mvalue) {
String[] Mstr = Mstrold.split(",");
switch (mno){
case M1:
Mstr[0]=String.valueOf(Mvalue);
break;
case M2:
Mstr[1]=String.valueOf(Mvalue);
break;
case M3:
Mstr[2]=String.valueOf(Mvalue);
break;
case M4:
Mstr[3]=String.valueOf(Mvalue);
break;
case M5:
Mstr[4]=String.valueOf(Mvalue);
break;
case M6:
Mstr[5]=String.valueOf(Mvalue);
break;
}
Mstrold=Mstr[0] + "," + Mstr[1] + "," + Mstr[2] + "," + Mstr[3] + "," + Mstr[4] + "," + Mstr[5];
String str = "s" + Mstrold + ";";
//文字列を送信する
byte[] bytes_ = {};
bytes_ = str.getBytes();
try {
//ここで送信
mOutput.write(bytes_);
} catch (IOException e) {
try {
mBtSocket.close();
} catch (IOException e1) {/*ignore*/}
}
}
@Override
protected void onDestroy() {
super.onDestroy();
// ソケットを閉じる
try {
mBtSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:gravity="center_vertical|center_horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/displayView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M1textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M1LabelView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="M1 "
android:textColor="@color/design_default_color_error"
android:textSize="36sp" />
<com.google.android.material.slider.Slider
android:id="@+id/M1slider"
android:layout_width="400dp"
android:layout_height="wrap_content"
android:stepSize="15.0"
android:value="0.0"
android:valueFrom="0.0"
android:valueTo="180.0" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M2textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M2LabelView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="M2 "
android:textColor="@color/design_default_color_error"
android:textSize="36sp" />
<com.google.android.material.slider.Slider
android:id="@+id/M2slider"
android:layout_width="400dp"
android:layout_height="wrap_content"
android:stepSize="15.0"
android:value="135.0"
android:valueFrom="15.0"
android:valueTo="165.0" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M3textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M3LabelView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="M3 "
android:textColor="@color/design_default_color_error"
android:textSize="36sp" />
<com.google.android.material.slider.Slider
android:id="@+id/M3slider"
android:layout_width="400dp"
android:layout_height="wrap_content"
android:stepSize="15.0"
android:value="0.0"
android:valueFrom="0.0"
android:valueTo="180.0" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M4textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M4LabelView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="M4 "
android:textColor="@color/design_default_color_error"
android:textSize="36sp" />
<com.google.android.material.slider.Slider
android:id="@+id/M4slider"
android:layout_width="400dp"
android:layout_height="wrap_content"
android:stepSize="15.0"
android:value="0.0"
android:valueFrom="0.0"
android:valueTo="180.0" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M5textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M5LabelView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="M5 "
android:textColor="@color/design_default_color_error"
android:textSize="36sp" />
<com.google.android.material.slider.Slider
android:id="@+id/M5slider"
android:layout_width="400dp"
android:layout_height="wrap_content"
android:stepSize="15.0"
android:value="0.0"
android:valueFrom="0.0"
android:valueTo="180.0" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M6textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp" />
</LinearLayout>
<LinearLayout
android:layout_width="500dp"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/M6LabelView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="M6 "
android:textColor="@color/design_default_color_error"
android:textSize="36sp" />
<com.google.android.material.slider.Slider
android:id="@+id/M6slider"
android:layout_width="400dp"
android:layout_height="wrap_content"
android:stepSize="5.0"
android:value="0.0"
android:valueFrom="0.0"
android:valueTo="70.0" />
</LinearLayout>
</LinearLayout>